Delivery Integration
Overview
iDB communicates with your item shop site via REST API calls. At the bare minimum, your website will need to…- ... expose order data via a GET endpoint which will be polled every X seconds
- ... accept order results via a POST endpoint
Exposing active orders
- This API should be a GET.
- Should return a list of currently active orders.
- Example URL might be https://myitemshop.com/api/get.php?pass=woiuxljkaoq123.
<?xml version="1.0" encoding="iso-8859-1"?>
<data>
<orders>
<order id="4000623">
<product sku="4000000" count="1" />
<product sku="4000001" count="5" />
</order>
<order id="4000605">
<product sku="4001003" count="1" />
</order>
</orders>
</data>
or JSON format:
{
"orders": [
{
"id": "4000623",
"products": [
{
"sku": "4000000",
"count": "1"
},
{
"sku": "4000001",
"count": "5"
}
]
},
{
"id": "4000624",
"products": [
{
"sku": "4001003",
"count": "1"
}
]
}
]
}
Receiving updated order data
- This API should be a POST.
- Should update order status and if state is 'Done' then send customer email containing mule accounts.
- extra field should be added to order notes.
- Example URL might be https://myitemshop.com/api/post.php?pass=woiuxljkaoq123.
<orders>
<order id="12345">
<account name="account1" password="password" />
<account name="account2" password="password" />
<state>Failed</state>
<missing>
<product sku="2006392" count="1" />
<product sku="2004323" count="2" />
</missing>
<extra>
Missing:
1×2006392-Call To Arms Crystal Sword – +6 BO
2×2004323-Immortal King's Soul Cage
Took from John:
1×2001822-Barbarian Hellfire 17-19 Resist All/17-19 Stats
1×2004323-Immortal King's Soul Cage
Took from David:
1×2001822-Barbarian Hellfire 17-19 Resist All/17-19 Stats
</extra>
</order>
<order id="99999">
<account name="account3" password="password" />
<account name="account4" password="password" />
<state>Done</state>
<extra>
Took from John:
1×2001822-Barbarian Hellfire 17-19 Resist All/17-19 Stats
1×2004323-Immortal King's Soul Cage
Took from David:
1×2001822-Barbarian Hellfire 17-19 Resist All/17-19 Stats
</extra>
</order>
<order id="98765">
<account name="account5" password="password" />
<account name="account6" password="password" />
<state>Done</state>
</order>
</orders>
or JSON format:
{
"orders": [
{
"id": 12345,
"state": "Failed",
"accounts": [
{
"name": "account1",
"password": "password"
},
{
"name": "account2",
"password": "password"
}
],
"missing": [
{
"sku": "2006392",
"count": 1
},
{
"sku": "2004323",
"count": 2
}
],
"extra": "Missing:\n1×2006392-Call To Arms Crystal Sword – +6 BO\n2×2004323-Immortal King's Soul Cage\nTook from John:\n1×2001822-Barbarian Hellfire 17-19 Resist All/17-19 Stats\n1×2004323-Immortal King's Soul Cage\nTook from David:\n1×2001822-Barbarian Hellfire 17-19 Resist All/17-19 Stats"
},
{
"id": 99999,
"state": "Done",
"accounts": [
{
"name": "account3",
"password": "password"
},
{
"name": "account4",
"password": "password"
}
],
"extra": "Took from John:\n1×2001822-Barbarian Hellfire 17-19 Resist All/17-19 Stats\n1×2004323-Immortal King's Soul Cage\nTook from David:\n1×2001822-Barbarian Hellfire 17-19 Resist All/17-19 Stats"
},
{
"id": 98765,
"state": "Done",
"accounts": [
{
"name": "account5",
"password": "password"
},
{
"name": "account6",
"password": "password"
}
]
}
]
}
SKU Design
Terminology:- Realm is a combination of server, core, ladder (eg. east softcore non-ladder, west hardcore ladder, etc)
- Code is similar to an SKU except it is realm-less (eg. Mara's Kaleidoscope 20-24 Resist All)
Realm | From | To |
---|---|---|
EuSCL | 1000000 | 1999999 |
EuSCNL | 2000000 | 2999999 |
EuSCCL | 3000000 | 3999999 |
EuSCCNL | 4000000 | 4999999 |
EuHCL | 5000000 | 5999999 |
EuHCNL | 6000000 | 6999999 |
EuHCCL | 7000000 | 7999999 |
EuHCCNL | 8000000 | 8999999 |
ESCL | 9000000 | 9999999 |
ESCNL | 10000000 | 10999999 |
ESCCL | 11000000 | 11999999 |
ESCCNL | 12000000 | 12999999 |
EHCL | 13000000 | 13999999 |
EHCNL | 14000000 | 14999999 |
EHCCL | 15000000 | 15999999 |
EHCCNL | 16000000 | 16999999 |
WSCL | 17000000 | 17999999 |
WSCNL | 18000000 | 18999999 |
WSCCL | 19000000 | 19999999 |
WSCCNL | 20000000 | 20999999 |
WHCL | 21000000 | 21999999 |
WHCNL | 22000000 | 22999999 |
WHCCL | 23000000 | 23999999 |
WHCCNL | 24000000 | 24999999 |
ASCL | 25000000 | 25999999 |
ASCNL | 26000000 | 26999999 |
ASCCL | 27000000 | 27999999 |
ASCCNL | 28000000 | 28999999 |
AHCL | 29000000 | 29999999 |
AHCNL | 30000000 | 30999999 |
AHCCL | 31000000 | 31999999 |
AHCCNL | 32000000 | 32999999 |
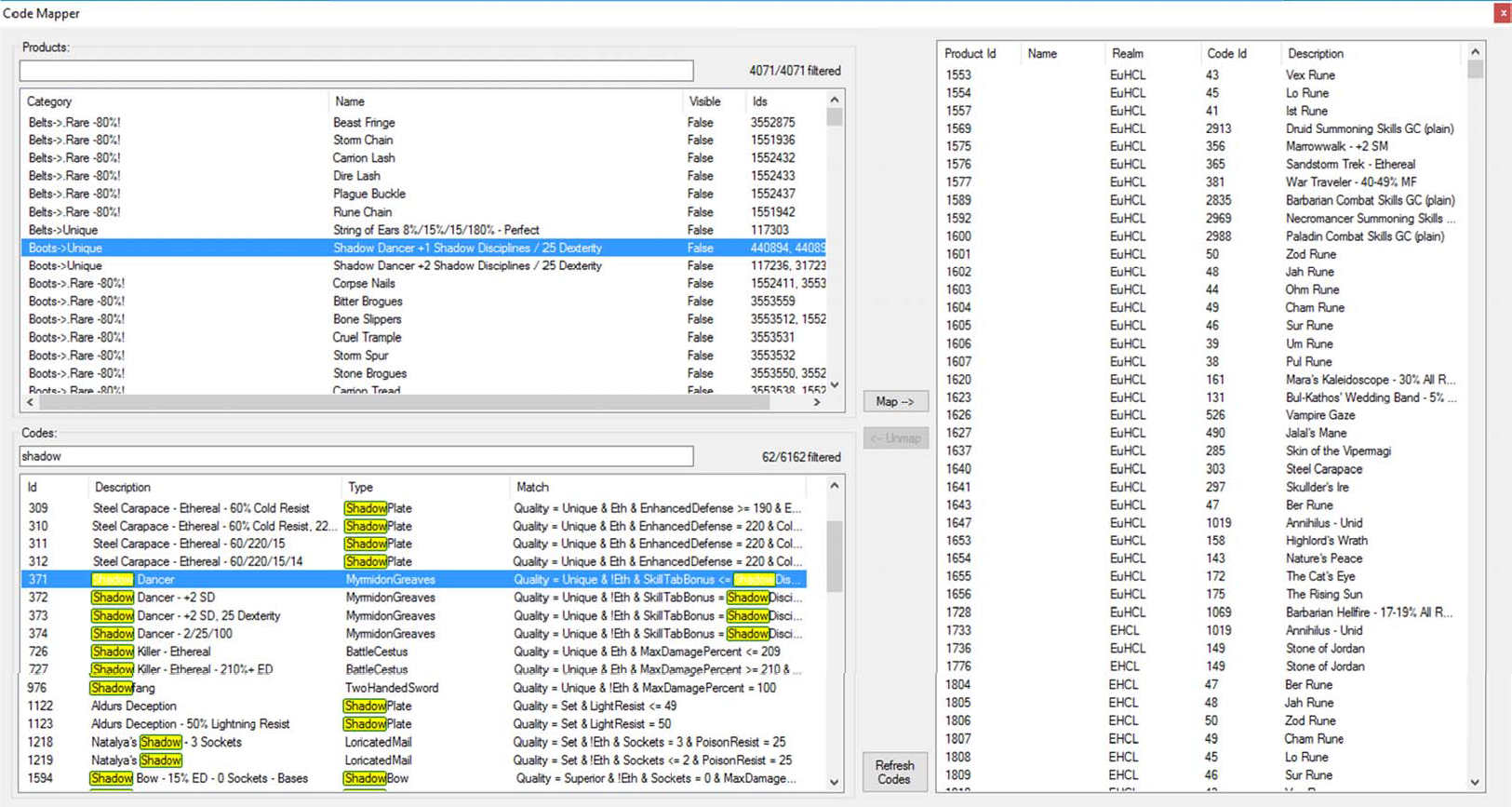
On top is the web shop products export from csv. iDB parses it and combines where possible (like if you have same item category/name for each realm). For example, you can see Shadow Dancer has couple IDs there. That means each of those IDs is from different realm and the mapper detected that and grouped them together.
Each product needs to be mapped. For example, select Shadow Dancer from top products and then search the iDB codes on bottom with the filter. Select the correct one, then hit the map button in the middle.
After done, for deliveries, your web shop can just send your product ids/skus and iDB will map over to iDB's codes. Also, you don't have to map all products. You can leave some products unmapped and then order will just partially complete and you can manually fulfill those products that failed due to missing mapping.
Quick publishing magics/rares
You will expose a new API endpoint on your item shop that accepts POSTs of JSON data. 3 different types of requests need to be handled: create, update, delete. Alternatively you could expose 3 different endpoints.Create
{
"type": "create",
"hash": "H-ASCNL-9FC067264C4F3DB59F3D4AAED55C8972CA0342C86DA4E7E525780E8B31855D89",
"realm": "ASCNL",
"image": "https://i.imgur.com/KYzpUEe.png",
"thumb": "https://i.imgur.com/U0TW73m.png",
"quality": "Rare",
"base": "Ring",
"kind": "Ring",
"graphic": "Orange",
"levelRequirement": 60,
"title": "Beast Circle",
"description": "<font color='#e6e200'>Beast Circle (95)<br/>Ring<br/></font><font color='#ff4d4d'>Required Level: 60<br/></font><font color='#6969ff'>+10% Faster Cast Rate<br/>5% Mana stolen per hit<br/>+37 to Life<br/>Fire Resist +19%<br/>Poison Resist +28%<br/>",
"price": 3.0,
"count": 1
}
- hash can be assumed to be fully unique and treated like an SKU, meaning you can send it in an order.
- realm syntax is [W/E/Eu/W][SC/HC][C/][L/NL] for a total of 32 possibilities.
- image and thumb links will not be hosted on Imgur but instead will look like https://*.transcendsharp.com/Search/GetItemImage?hash={hash}&signature={hashSignature}, you should download and host the image yourself if you intend to show it to customers.
- quality sourced from https://diablo.transcendsharp.com/content/game-data/Qualities.txt
- base sourced from https://diablo.transcendsharp.com/content/game-data/Types.txt
- kind sourced from https://diablo.transcendsharp.com/content/game-data/Kinds.txt
- graphic sourced from https://diablo.transcendsharp.com/content/game-data/Graphics.txt
-
Upon request, variations can be made:
- Using base-64 encoded PNG data instead of links for the images. This increases payload size substantially but saves you the extra work of going to download the images separately.
- Adding a categoryId property with custom mapping logic based on item quality & kind.
- Substituting colors in the description HTML to fit color scheme of your site.
- Excluding title or base name from description HTML.
- .. and many more
Update
{
"type": "update",
"hash": "H-ASCNL-9FC067264C4F3DB59F3D4AAED55C8972CA0342C86DA4E7E525780E8B31855D89",
"count": 5
}
This means you should already have the product details; we are just sending an updated count.
Delete
{
"type": "delete",
"hash": "H-ASCNL-9FC067264C4F3DB59F3D4AAED55C8972CA0342C86DA4E7E525780E8B31855D89"
}
This means you should delete the product, it's no longer available.
Ladder reset handling
A variety of approaches can be taken...- Easiest approach is to untrack all ladder items. iDB can notify your item shop that each product should be deleted.
- Next approach is to untrack all ladder items locally only. In your item shop you would need to build & run some script that deletes all the tracked ladder items. This would be very fast.
- Next approach is to have iDB untrack all ladder items and re-track them on non-ladder. This can be very time consuming if you have a lot of items tracked and your item shop might end up re-downloading images, etc.
- Next approach is to have iDB untrack all ladder items and re-track them on non-ladder locally only. In your item shop you would need to build & run some script that updates all the hashes to the appropriate non-ladder variant (eg. ESCL -> ESCNL). This would be very fast.
Updating product in-stock counts
Not yet documented.Changelog
2021-02-04:
- ADD Initial